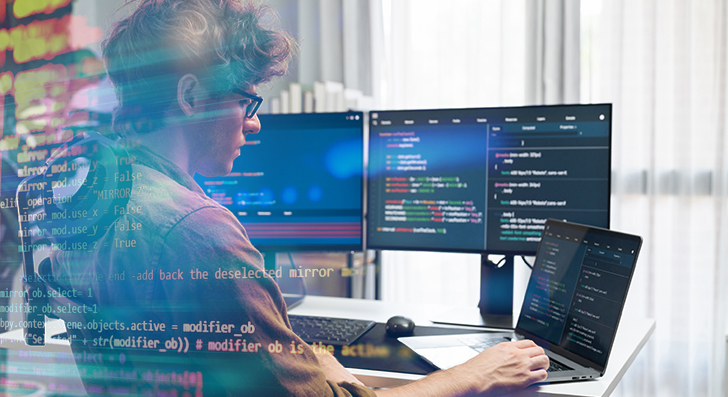
Scalability implies your software can take care of development—more buyers, additional info, and even more visitors—without the need of breaking. For a developer, creating with scalability in mind will save time and tension afterwards. Listed here’s a transparent and functional manual to assist you to start off by Gustavo Woltmann.
Style for Scalability from the beginning
Scalability is not a little something you bolt on later on—it ought to be element within your program from the start. Several purposes fall short every time they expand speedy since the first design and style can’t tackle the extra load. Being a developer, you need to Consider early regarding how your program will behave stressed.
Start by developing your architecture to generally be flexible. Keep away from monolithic codebases where every little thing is tightly related. Instead, use modular layout or microservices. These styles crack your app into more compact, impartial pieces. Every module or provider can scale By itself without affecting The entire process.
Also, think about your database from day one particular. Will it will need to take care of a million customers or perhaps 100? Select the suitable type—relational or NoSQL—according to how your info will increase. System for sharding, indexing, and backups early, Even when you don’t have to have them yet.
An additional crucial level is in order to avoid hardcoding assumptions. Don’t publish code that only will work underneath present-day disorders. Think about what would happen if your person foundation doubled tomorrow. Would your application crash? Would the database slow down?
Use style patterns that support scaling, like message queues or party-pushed devices. These enable your application take care of far more requests without having acquiring overloaded.
Once you Develop with scalability in mind, you are not just planning for achievement—you are decreasing future problems. A very well-prepared method is easier to take care of, adapt, and increase. It’s far better to organize early than to rebuild afterwards.
Use the appropriate Database
Choosing the ideal databases is actually a important Element of making scalable programs. Not all databases are built a similar, and utilizing the Incorrect you can sluggish you down or even induce failures as your app grows.
Start by knowledge your knowledge. Is it remarkably structured, like rows within a desk? If Indeed, a relational databases like PostgreSQL or MySQL is an efficient match. These are definitely sturdy with relationships, transactions, and regularity. They also assist scaling methods like examine replicas, indexing, and partitioning to deal with additional site visitors and details.
Should your details is much more adaptable—like user action logs, product catalogs, or paperwork—take into consideration a NoSQL alternative like MongoDB, Cassandra, or DynamoDB. NoSQL databases are improved at handling substantial volumes of unstructured or semi-structured data and might scale horizontally extra very easily.
Also, look at your study and publish styles. Are you currently executing lots of reads with fewer writes? Use caching and browse replicas. Are you presently handling a large produce load? Look into databases that will cope with superior create throughput, as well as celebration-centered information storage devices like Apache Kafka (for temporary information streams).
It’s also wise to Consider in advance. You might not have to have Sophisticated scaling functions now, but selecting a database that supports them suggests you received’t have to have to modify afterwards.
Use indexing to hurry up queries. Avoid unnecessary joins. Normalize or denormalize your information according to your accessibility designs. And often keep an eye on database functionality while you increase.
In a nutshell, the best databases is dependent upon your app’s construction, speed requirements, and how you expect it to grow. Choose time to select correctly—it’ll help save a great deal of problems later.
Optimize Code and Queries
Fast code is essential to scalability. As your application grows, every single tiny delay provides up. Inadequately prepared code or unoptimized queries can slow down overall performance and overload your system. That’s why it’s imperative that you Establish successful logic from the start.
Start by crafting clean, straightforward code. Stay clear of repeating logic and remove just about anything unnecessary. Don’t pick the most intricate Option if an easy one functions. Keep the features brief, concentrated, and simple to test. Use profiling tools to locate bottlenecks—sites wherever your code will take also extensive to operate or makes use of an excessive amount of memory.
Future, check out your database queries. These frequently gradual items down greater than the code alone. Be sure Every question only asks for the data you really need. Keep away from SELECT *, which fetches all the things, and as an alternative find precise fields. Use indexes to speed up lookups. And stay away from accomplishing too many joins, In particular across substantial tables.
In case you notice exactly the same facts becoming requested again and again, use caching. Retailer the effects briefly using instruments like Redis or Memcached therefore you don’t need to repeat high priced functions.
Also, batch your database operations any time you can. Instead of updating a row one by one, update them in groups. This cuts down on overhead and would make your application more productive.
Make sure to test with huge datasets. Code and queries that get the job done good with a hundred documents might crash after they have to take care of one million.
In short, scalable apps are quickly apps. Keep the code limited, your queries lean, and use caching when required. These measures support your software stay clean and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your application grows, it's got to handle much more consumers and a lot more website traffic. If anything goes as a result of a person server, it will eventually immediately turn into a bottleneck. That’s wherever load balancing and caching can be found in. Both of these equipment support maintain your app quick, stable, and scalable.
Load balancing spreads incoming targeted visitors throughout a number of servers. As an alternative to a single server carrying out each of the function, the load balancer routes users to distinctive servers dependant on availability. What this means is no solitary server gets overloaded. If a person server goes down, the load balancer can send out traffic to the Other people. Tools like Nginx, HAProxy, or cloud-dependent remedies from AWS and Google Cloud make this simple to set up.
Caching is about storing details briefly so it can be reused immediately. When people request the same facts once again—like a product web site or possibly a profile—you don’t have to fetch it within the databases each time. You could serve it from the cache.
There are 2 typical different types of caching:
1. Server-facet caching (like Redis or Memcached) retailers data in memory for rapidly access.
two. Client-aspect caching (like browser caching or CDN caching) stores static documents near the consumer.
Caching cuts down database load, increases speed, and would make your app far more efficient.
Use caching for things that don’t improve usually. And normally ensure your cache is current when info does transform.
In brief, load balancing and caching are basic but powerful equipment. Alongside one another, they help your application tackle much more end users, stay quickly, and Get well from problems. If you plan to increase, you would like the two.
Use Cloud and Container Instruments
To make scalable programs, you may need applications that let your app expand quickly. That’s where by cloud platforms and containers come in. They offer you adaptability, reduce setup time, and make scaling Significantly smoother.
Cloud platforms like Amazon World-wide-web Products and services (AWS), Google Cloud System (GCP), and Microsoft Azure let you rent servers and solutions as you will need them. You don’t really need to obtain components or guess upcoming potential. When traffic increases, you are able to include a lot more assets with only a few clicks or routinely employing automobile-scaling. When targeted traffic drops, it is possible to scale down to save cash.
These platforms also supply providers like managed databases, storage, load balancing, and safety resources. You'll be able to give attention to creating your app instead of managing infrastructure.
Containers are A further vital Resource. A container deals your app and everything it needs to operate—code, libraries, options—into a single unit. This can make it uncomplicated to move your app concerning environments, from the laptop to the cloud, without the need of surprises. Docker is the preferred Device for this.
When your application employs numerous containers, applications like Kubernetes make it easier to deal with them. Kubernetes handles deployment, scaling, and recovery. If just one portion of one's application crashes, it restarts it routinely.
Containers also make it straightforward to independent parts of your application into solutions. You could update or scale areas independently, which is perfect for overall performance and trustworthiness.
In brief, using cloud and container equipment means you may scale quick, deploy quickly, and Recuperate promptly when difficulties transpire. If you'd like your application to develop devoid of limits, start off using these equipment early. They help you save time, decrease possibility, and help you remain centered on building, not repairing.
Watch Every thing
In case you don’t observe your application, you gained’t know when points go wrong. Monitoring will help the thing is how your application is carrying out, place troubles early, and make improved decisions as your app grows. It’s a essential Element of building scalable techniques.
Start off by monitoring essential metrics like CPU use, memory, disk space, and response time. These let you know how your servers and companies are executing. Applications like Prometheus, Grafana, Datadog, or New Relic can help you gather and visualize this info.
Don’t just keep an eye on your servers—watch your application far too. Regulate how much time it's going to take for users to load pages, how often errors happen, and exactly where they happen. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will help you see what’s taking place inside here your code.
Setup alerts for essential issues. For instance, In case your response time goes above a Restrict or simply a assistance goes down, it is best to get notified promptly. This will help you correct concerns quickly, frequently prior to users even see.
Checking can be beneficial whenever you make changes. For those who deploy a different attribute and see a spike in errors or slowdowns, you may roll it back again prior to it causes authentic hurt.
As your app grows, targeted visitors and knowledge improve. Without checking, you’ll skip indications of problems until it’s far too late. But with the best tools set up, you stay on top of things.
In brief, checking aids you keep the app reliable and scalable. It’s not almost recognizing failures—it’s about comprehension your method and making certain it works very well, even under pressure.
Closing Thoughts
Scalability isn’t only for huge providers. Even tiny applications require a robust foundation. By planning carefully, optimizing properly, and utilizing the correct instruments, you can Create applications that develop efficiently without breaking under pressure. Start out small, Consider significant, and Develop clever.